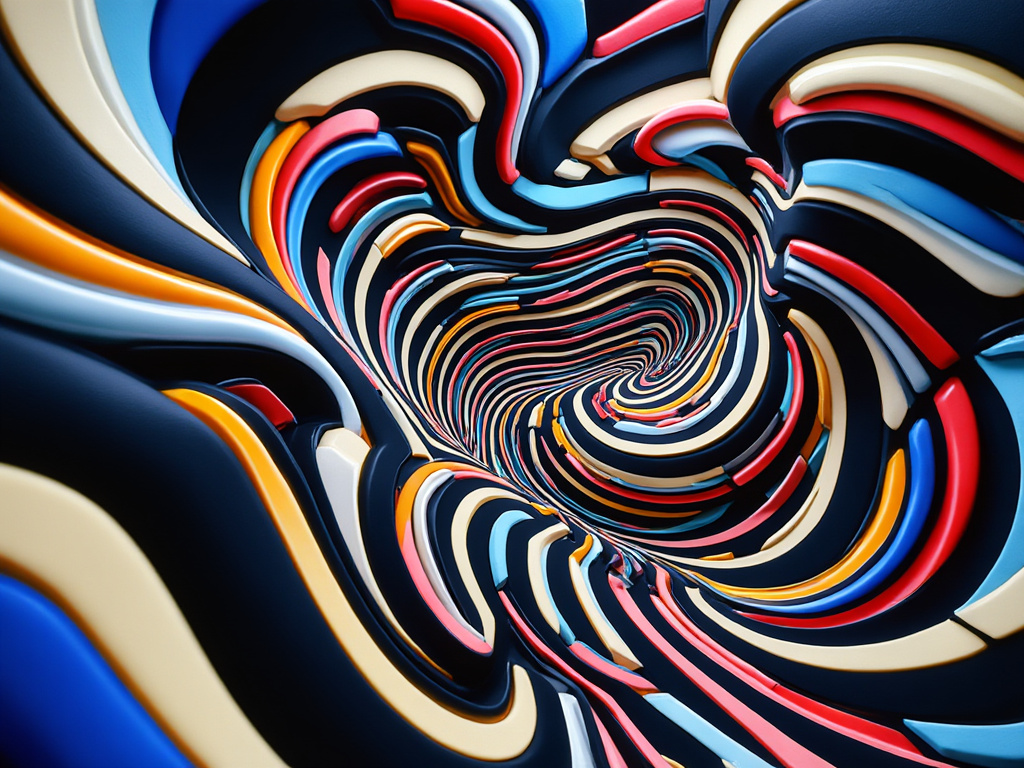
Model Quantization Across Providers
A deep dive into model quantization techniques and their implementation across different ML providers.
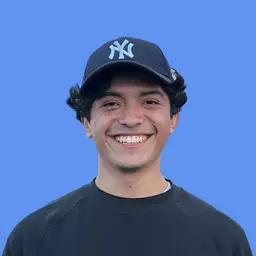
Disclaimer: The views and opinions expressed in this blog are my own and do not necessarily reflect the official position of my employer.
Model quantization is a crucial technique in machine learning deployment that reduces model size and improves inference speed while maintaining acceptable accuracy. In this deep dive, we'll explore how quantization works, its benefits, and how different providers implement it.
Understanding Quantization
At its core, quantization is the process of mapping values from a large set (such as floating-point numbers) to values in a smaller set (such as 8-bit integers). This process is similar to reducing the color depth of an image - while some precision is lost, the essential information remains intact while significantly reducing the storage requirements.
Types of Quantization
Benefits of Quantization
Implementation Across Providers
Provider | Techniques | Precision |
---|---|---|
TensorFlow Lite | Post-training, Aware-training | INT8, FP16 |
PyTorch | Dynamic, Static | INT8, FP16 |
ONNX Runtime | Post-training | INT8 |
Bit-Level Representation
This visualization demonstrates how floating-point numbers are converted to reduced-precision integers during quantization, showing the actual bit-level transformations that occur.
BrainFloat16 vs Standard FP16
While both BF16 and FP16 use 16 bits, they allocate these bits differently:
- FP16 (Half Precision): 1 sign bit, 5 exponent bits, 10 mantissa bits
- BF16 (BrainFloat16): 1 sign bit, 8 exponent bits, 7 mantissa bits
BF16 maintains the same dynamic range as FP32 (due to 8 exponent bits) but trades precision for better numerical stability in deep learning applications. This makes it particularly suitable for training neural networks.
Best Practices
- Start with post-training quantization for its simplicity
- Measure accuracy impact on a representative validation set
- Consider quantization-aware training if accuracy loss is unacceptable
- Profile your model to identify performance bottlenecks
- Test on target hardware to ensure expected performance gains
Quick Implementation Example
# TensorFlow Lite Quantization Example
import tensorflow as tf
# Convert to TF Lite model
converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_path)
converter.optimizations = [tf.lite.Optimize.DEFAULT]
converter.target_spec.supported_types = [tf.int8]
# Enable quantization
converter.representative_dataset = representative_dataset_gen
quantized_tflite_model = converter.convert()
Frequently Asked Questions
What is model quantization in machine learning?
Model quantization is a technique that reduces the precision of numbers used in a neural network, converting them from 32-bit floating-point to lower-precision formats like 8-bit integers, resulting in smaller model sizes and faster inference while maintaining acceptable accuracy.
What are the main benefits of model quantization?
The main benefits include reduced model size (up to 75% smaller), faster inference speed, lower memory bandwidth requirements, and improved energy efficiency, making models more suitable for deployment on edge devices and mobile applications.
Which quantization technique should I use for my ML model?
For beginners, post-training quantization is recommended due to its simplicity. If accuracy degradation is too high, consider quantization-aware training. The choice depends on your accuracy requirements, deployment constraints, and target hardware capabilities.